Posted on: September 08, 2021 09:52 AM
Posted by: Renato
Views: 1032
Laravel upload arquivos para DropBox gratuito
Requisitos
PHP versão 7.1 ou 7.2 ou 7. +
Vamos criar / instalar o Laravel mais recente através do #CLI com o seguinte comando no diretório do seu projeto.
|
|
Após a instalação do Laravel Framework instale
Instale o pacote de driver do Laravel Dropbox
Aqui iremos através do #CLI instalar o pacote para comunicar a caixa de depósito. Abra o comando / cli em seu diretório Laravel instalado e execute o seguinte comando
composer require spatie/flysystem-dropbox
Criar aplicativo e gerar token de acesso
Registre a conta ou faça login para criar o aplicativo e gerar o token de acesso à caixa de depósito a partir do seguinte url https://www.dropbox.com/developers/apps
Preencha os campos a seguir e clique no botão criar aplicativo.
Gerar token de acesso do aplicativo Dropbox
Vá para o seu aplicativo e clique em gerar para gerar token de acesso. mantenha esse token em seu arquivo que definiremos no arquivo .env na próxima etapa.
dropbox-generate-access-token-button
Definir token no arquivo .env
|
|
Integrar / configurar benjamincrozat / laravel-dropbox-driver
Incluindo provedores em config / app .file
App\Providers\DropboxServiceProvider::class,
Adicionar a seguinte matriz do Dropbox às configurações de disco em config / filesystem.php
|
|
Criar controlador
Crie o arquivo do controlador DropboxController.php no diretório app / Http / Controllers com o seguinte código
|
|
Definir rota para upload
Escreva as seguintes linhas no arquivo web.php para a rota de upload do arquivo no Dropbox
|
|
Criar vista
Vamos criar um arquivo de visão no diretório resources \ views e nomeá- lo “dropbox.blade.php” aqui vamos usar #bladeTemplate
Escreva o seguinte código no arquivo
|
|
Acesse-o em seu host local com o seguinte url
localhost / laraveldropbox / public / upload-to-dropbox /
laravel remover público do url
Criar rota para mão / upload de arquivo
Escreva a seguinte linha em seu arquivo de roteamento routes / web.php para manipular quando o arquivo for carregado e enviado ao seu servidor.
|
|
Adicionar espaços de nomes no arquivo DropboxController
|
|
Criar método para manuseio e upload de arquivo
Método de Criação
|
|
Nota:
Custom Filesystems
Laravel's Flysystem integration provides support for several "drivers" out of the box; however, Flysystem is not limited to these and has adapters for many other storage systems. You can create a custom driver if you want to use one of these additional adapters in your Laravel application.
In order to define a custom filesystem you will need a Flysystem adapter. Let's add a community maintained Dropbox adapter to our project:
composer require spatie/flysystem-dropbox
Next, you can register the driver within the boot
method of one of your application's service providers. To accomplish this, you should use the extend
method of the Storage
facade:
<?php
namespace App\Providers;
use Illuminate\Support\Facades\Storage;
use Illuminate\Support\ServiceProvider;
use League\Flysystem\Filesystem;
use Spatie\Dropbox\Client as DropboxClient;
use Spatie\FlysystemDropbox\DropboxAdapter;
class AppServiceProvider extends ServiceProvider
{
/**
* Register any application services.
*
* @return void
*/
public function register()
{
//
}
/**
* Bootstrap any application services.
*
* @return void
*/
public function boot()
{
Storage::extend('dropbox', function ($app, $config) {
$client = new DropboxClient(
$config['authorization_token']
);
return new Filesystem(new DropboxAdapter($client));
});
}
}
The first argument of the extend
method is the name of the driver and the second is a closure that receives the $app
and $config
variables. The closure must return an instance of League\Flysystem\Filesystem
. The $config
variable contains the values defined in config/filesystems.php
for the specified disk.
Once you have created and registered the extension's service provider, you may use the dropbox
driver in your config/filesystems.php
configuration file.
DropboxFilesystemServiceProvider like this:
<?php
namespace App\Providers;
use Storage;
use League\Flysystem\Filesystem;
use Dropbox\Client as DropboxClient;
use League\Flysystem\Dropbox\DropboxAdapter;
use Illuminate\Support\ServiceProvider;
class DropboxFilesystemServiceProvider extends ServiceProvider
{
public function boot()
{
Storage::extend('dropbox', function ($app, $config) {
$client = new DropboxClient($config['accessToken'], $config['appSecret']);
return new Filesystem(new DropboxAdapter($client));
});
}
public function register()
{
//
}
}
The service provider is also added to my config/app providers:
'providers' => [
...
App\Providers\DropboxServiceProvider::class,
...
]
In my config/filesystems I've added the dropbox driver (dropbox app key and secret are also set in .env file):
'dropbox' => [
'driver' => 'dropbox',
'key' => env('DROPBOX_APP_KEY'),
'secret' => env('DROPBOX_APP_SECRET'),
]
Now, when I try to run the following code, it returns false and the file doesn't appear in my Dropbox. When I change the disk to 'local', the file gets uploaded to my local storage.
$path = "pdf/file.pdf";
$storage_path = Storage::path($path);
$contents = file_get_contents($storage_path);
$upload = Storage::disk('dropbox')->put($path, $contents);
return $upload;
https://laravel.com/docs/8.x/filesystem
https://github.com/spatie/flysystem-dropbox
https://www.youtube.com/watch?v=MEhibvy31oI
https://github.com/LaravelDaily/Laravel-File-Storage
https://flysystem.thephpleague.com/v1/docs/adapter/dropbox/
Donate to Site
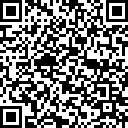
Renato
Developer