Posted on: April 07, 2025 03:25 PM
Posted by: Renato
Categories: Laravel
Views: 38
Eloquent Fill and Insert Method in Laravel 12.6
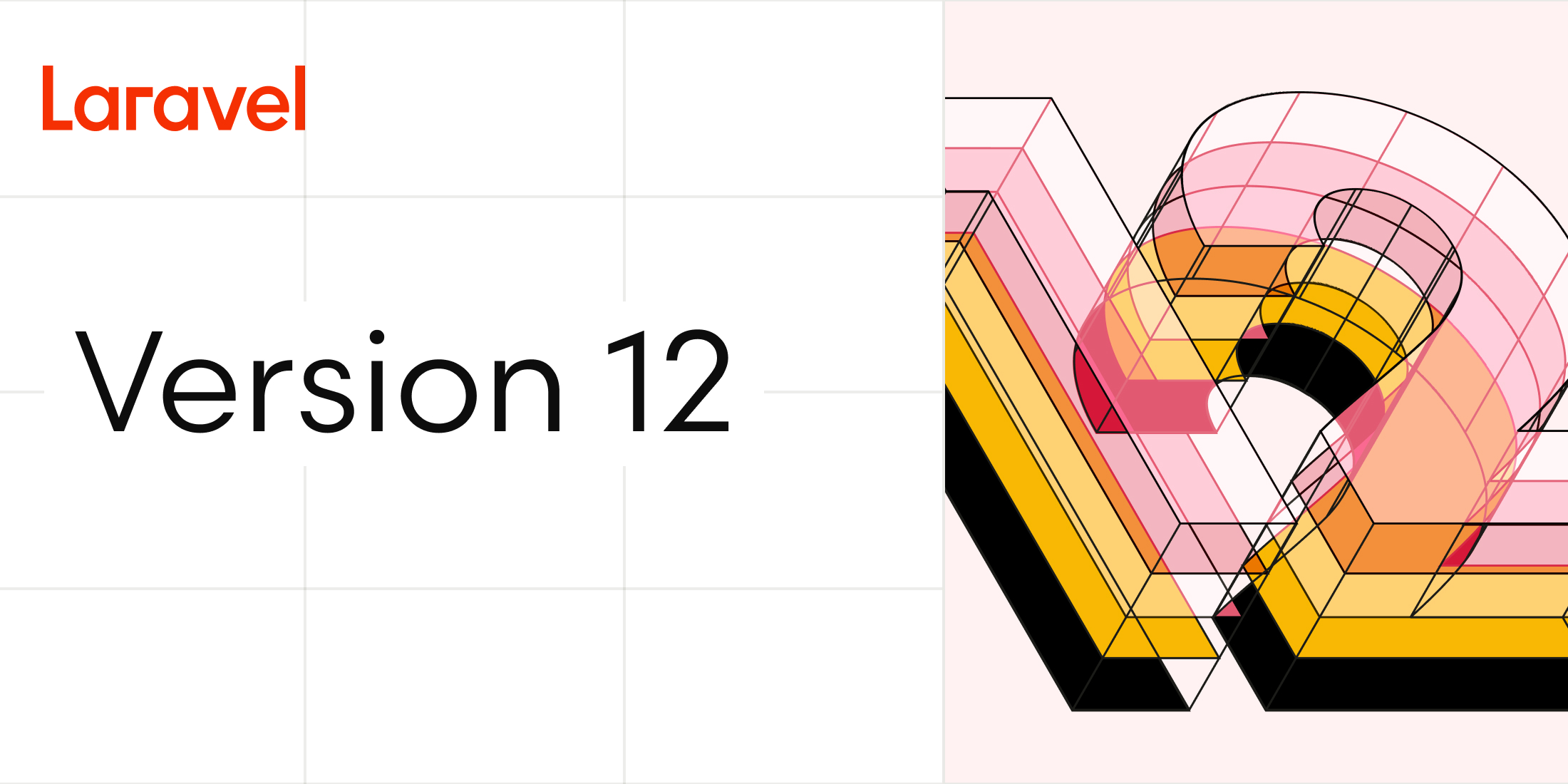
The Laravel team released v12.6.0, which includes an Eloquent fillAndInsert()
method, a URI path segments helper, a Password appliedRules()
method, and more.
Allow Merging Model Attributes Before Insert
Luke Kuzmish contributed a fillAndInsert()
method to "manually cast values to primitives, set timestamps, or set UUIDs":
ModelWithUniqueStringIds::fillAndInsert([ [ 'name' => 'Taylor', 'role' => IntBackedRole::Admin, 'role_string' => StringBackedRole::Admin, ], [ 'name' => 'Nuno', 'role' => 3, 'role_string' => 'admin', ], [ 'name' => 'Dries', 'uuid' => 'bbbb0000-0000-7000-0000-000000000000', ], [ 'name' => 'Chris', ],]);
See Pull Request #55038 for details.
Add HTTP Request Exception
Luke Kuzmish contributed a requestException()
method to use when you manually need to stub out an exception for the HTTP client:
// Before$exception = new RequestException( new Response( Http::response(['code' => 'not_found', 404)->wait() )); // After$exception = Http::requestException(['code' => 'not_found'], 404);
See Pull Request #55241 for details.
URI Path Segments Helper Method
Chester Sykes contributed a new pathSegments()
method to the Uri
class that returns each part of the URI path as a collection of elements:
$uri = Uri::of('https://laravel.com/one/two/three'); // Manually$firstSegment = collect(explode('/', $uri->path()))->first(); // 'one' // Using the pathSegments() method$firstSegment = $uri->pathSegments()->first();
Password appliedRules Method
@devajmeireles contributed an appliedRules()
method to the Password
fluent rule builder. The appliedRules()
method returns an array of password validation rules and their usage statuses.
Given the following code to define your app's password rules:
Password::defaults(function () { return Password::min(8) ->mixedCase() ->numbers();}); // In a viewreturn view('livewire.profile', [ 'appliedRules' => Password::default()->appliedRules(),]);
<div> <form wire:submit="save"> <div> <x-input label="Password" /> <span>{{ __('The password must be at least :min characters long.', ['min' => $appliedRules['min']]) }}</span> @if ($appliedRules['mixedCase'] === true) <span>{{ __('The password must contain at least one uppercase and one lowercase letter.') }}</span> @endif @if ($appliedRules['numbers'] === true) <span>{{ __('The password must contain at least one number.') }}</span> @endif </div> <x-button type="submit"> Save </x-button> </form></div>
See Pull Request #55206 for implementation details.
db:seed
Command Prohibitable
Make the Benedikt Franke contributed the ability to make the SeedCommand
prohibitable in certain environments. The SeedCommand
is not prohibited when calling prohibitDestructiveCommands()
, however, after Pull Request #55238 you can call the following to prohibit the db:seed
command:
DB::prohibitDestructiveCommands();SeedCommand::prohibit();
Do Not Stop Pruning if One Model Fails
Günther Debrauwer contributed an update to the model:prune
command that continues running after an exception:
When you run model:prune command and an exception occurs during the pruning process of a single model, than the entire command will fail. I had this issue recently in a project. That meant no models were being pruned until I fixed the bug that prevented the pruning of a single model.
This PR fixes that. The model:prune command will skip the models that could not be pruned and report the exceptions that occurred when pruning those models.
See Pull Request #55237 for details.
Release notes
You can see the complete list of new features and updates below and the diff between 12.5.0 and 12.6.0 on GitHub. The following release notes are directly from the changelog:
v12.6.0
- [12.x] Dont stop pruning if pruning one model fails by @gdebrauwer in https://github.com/laravel/framework/pull/55237
- [12.x] Update Date Facade Docblocks by @fdalcin in https://github.com/laravel/framework/pull/55235
- Make
db:seed
command prohibitable by @spawnia in https://github.com/laravel/framework/pull/55238 - [12.x] Introducing
Rules\Password::appliedRules
Method by @devajmeireles in https://github.com/laravel/framework/pull/55206 - [12.x] Allowing merging model attributes before insert via
Model::fillAndInsert()
by @cosmastech in https://github.com/laravel/framework/pull/55038 - [12.x] Fix type hints for DateTimeZone and DateTimeInterface on DateFactory by @AndrewMast in https://github.com/laravel/framework/pull/55243
- [12.x] Fix DateFactory docblock type hints by @AndrewMast in https://github.com/laravel/framework/pull/55244
- List missing
migrate:rollback
in DB::prohibitDestructiveCommands PhpDoc by @spawnia in https://github.com/laravel/framework/pull/55252 - [12.x] Add
Http::requestException()
by @cosmastech in https://github.com/laravel/framework/pull/55241 - New: Uri
pathSegments()
helper method by @chester-sykes in https://github.com/laravel/framework/pull/55250 - [12.x] Do not require returning a Builder instance from a local scope method by @cosmastech in https://github.com/laravel/framework/pull/55246
Donate to Site
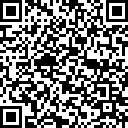
Renato
Developer